When dealing with online applications, it is typical to need to export HTML tables to CSV format. CSV is a popular format for exchanging data across programs because it is simple to read and interpret by software tools.
In this tutorial, we’ll teach you how to use jQuery to convert an HTML table to CSV format. The code samples supplied are merely for demonstration reasons, and you should modify them to fit your individual use case.
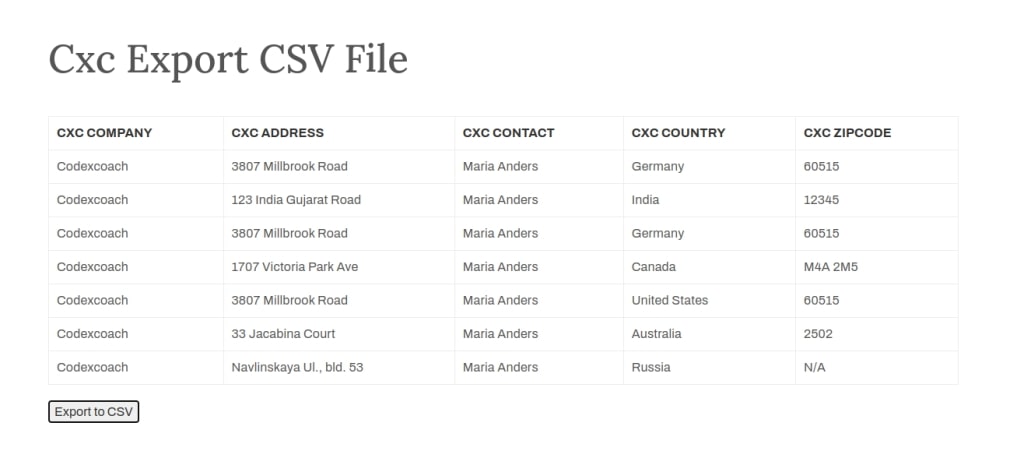
Step 1: Create an HTML table
We must first generate the HTML table that will be exported to CSV. A basic table is shown below.
<div class="cxc_main">
<table id="cxc_exp_table" class="cxc_exp_table" cellpadding="5" border="1" cellspacing="0">
<thead class="thead">
<tr class="th-list">
<th class="cxc-th-company">Cxc Company</th>
<th class="cxc-th-address">Cxc Address</th>
<th class="cxc-th-contact">Cxc Contact</th>
<th class="cxc-th-country">Cxc Country</th>
<th class="cxc-th-zipcode">Cxc Zipcode</th>
</tr>
</thead>
<tbody class="tbody">
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">3807 Millbrook Road</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">Germany</td>
<td class="cxc-zipcode">60515</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">123 India Gujarat Road</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">India</td>
<td class="cxc-zipcode">12345</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">3807 Millbrook Road</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">Germany</td>
<td class="cxc-zipcode">60515</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">1707 Victoria Park Ave</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">Canada</td>
<td class="cxc-zipcode">M4A 2M5</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">3807 Millbrook Road</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">United States</td>
<td class="cxc-zipcode">60515</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">33 Jacabina Court</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">Australia</td>
<td class="cxc-zipcode">2502</td>
</tr>
<tr class="tr-list">
<td class="cxc-company">Codexcoach</td>
<td class="cxc-address">Navlinskaya Ul., bld. 53</td>
<td class="cxc-contact">Maria Anders</td>
<td class="cxc-country">Russia</td>
<td class="cxc-zipcode">N/A</td>
</tr>
</tbody>
</table>
<div>
<button type="button" class="cxc_exp_to_csv" onclick="cxc_export_to_csv_file_fun()">Export to CSV</button>
</div>
</div>
Step 2: Write the JQuery code
we need to write the JQuery code that will handle the export process when the button is clicked. Here’s an example of how to do it:
<script type="text/javascript">
function cxc_export_to_csv_file_fun() {
var data_arr = [];
var th_arr = [];
if( jQuery('.cxc_exp_table .thead .th-list').length > 0 ){
jQuery('.cxc_exp_table .thead .th-list').each(function(){
var tr_this = jQuery(this);
var cxc_th_company = tr_this.find('.cxc-th-company').text();
th_arr.push( cxc_th_company );
var cxc_th_address = tr_this.find('.cxc-th-address').text();
th_arr.push( cxc_th_address );
var cxc_th_contact = tr_this.find('.cxc-th-contact').text();
th_arr.push( cxc_th_contact );
var cxc_th_country = tr_this.find('.cxc-th-country').text();
th_arr.push( cxc_th_country );
var cxc_th_zipcode = tr_this.find('.cxc-th-country').text();
th_arr.push( cxc_th_zipcode );
});
}
if( th_arr && th_arr.length > 0 ) {
data_arr.push( th_arr.join(",") );
}
if( jQuery('.cxc_exp_table .tbody .tr-list').length > 0 ){
jQuery('.cxc_exp_table .tbody .tr-list').each(function(){
var tr_this = jQuery(this);
var cxc_company = tr_this.find('.cxc-company').text();
cxc_company = cxc_company.trim();
var cxc_address = tr_this.find('.cxc-address').text();
cxc_address = cxc_address.trim();
var cxc_contact = tr_this.find('.cxc-contact').text();
cxc_contact = cxc_contact.trim();
var cxc_country = tr_this.find('.cxc-country').text();
cxc_country = cxc_country.trim();
var cxc_zipcode = tr_this.find('.cxc-country').text();
cxc_zipcode = cxc_zipcode.trim();
var data_obj = [
'"' + cxc_company + '"',
'"' + cxc_address + '"',
'"' + cxc_contact + '"',
'"' + cxc_country + '"',
'"' + cxc_zipcode + '"',
];
data_obj.map(string => string === null ? '' : `\"${string}\"`);
data_arr.push( data_obj.join(",") );
});
}
data_arr = data_arr.join('\n');
// return false;
// Create CSV file object and feed
// our csv_data into it
CSVFile = new Blob([data_arr], {
type: "text/csv"
});
// Create to temporary link to initiate
// download process
var temp_link = document.createElement('a');
// Download csv file
temp_link.download = "Cxc Export To CSV.csv";
var url = window.URL.createObjectURL(CSVFile);
temp_link.href = url;
// This link should not be displayed
temp_link.style.display = "none";
document.body.appendChild(temp_link);
// Automatically click the link to
// trigger download
temp_link.click();
document.body.removeChild(temp_link);
}
jQuery(document).ready( function() {
jQuery(document).on( 'click', '.cxc_exp_table .cxc_exp_to_csv', function () {
cxc_export_to_csv_file_fun();
} );
} );
</script>
Conclusion
We demonstrated how to use jQuery to convert an HTML table to CSV format. This solution is simple and straightforward to implement, and it can be tailored to different use cases by adjusting the HTML table and JavaScript code as needed.
FAQs
Why would I want to export an HTML table to CSV?
There are several reasons why you would wish to convert an HTML table to a CSV file. For example, you might offer users to download data from your online application in a format that can be readily accessed and analyzed in a spreadsheet program such as Microsoft Excel or Google Sheets.
Do I need to use jQuery to export an HTML table to CSV?
No, jQuery is not required to export an HTML table to CSV. jQuery, on the other hand, provides a straightforward way to modify the DOM and handle events in JavaScript, which helps simplify the exporting process.
How can I customize the CSV output format?
Because the CSV output format is defined by the data in the HTML table, you must adjust the table itself if you wish to change the format.
-
CodexCoach is a skilled tech educator known for easy-to-follow tutorials in coding, digital design, and software development. With practical tips and interactive examples, CodexCoach helps people learn new tech skills and advance their careers.
It’s really a cool and useful piece of information. I’m satisfied that you shared this useful information with us. Please keep us up to date like this. Thank you for sharing.
Oh, thank You!!! It’s fantastic! You helped me so much! Sorry for my English ))) I’m from Russia!