Creating custom product types in WooCommerce allows you to extend the functionality of your online store and provide unique shopping experiences to your customers.
By programmatically adding custom product types, you can define and customize products that are tailored to your specific business needs. In this guide, we will walk you through the process of creating a custom WooCommerce product type programmatically.
By following the steps outlined in this guide, you will learn how to create a custom product type and define its behavior, data fields, and display options. This process involves setting up a development environment, creating a custom plugin or modifying your theme’s functions.php file, and leveraging WooCommerce hooks and filters to register and customize your new product type.
Whether you want to create custom product types for specialized items, services, or unique offerings, this guide will equip you with the knowledge and tools to extend WooCommerce’s capabilities and create a tailored shopping experience for your customers. Let’s dive in and discover how to create a custom WooCommerce product type programmatically.
<?php
add_action( 'init', 'cxc_register_subscription_product_type' );
function cxc_register_subscription_product_type() {
class WC_Product_cxc_subscription extends WC_Product {
public function __construct( $product ) {
$this->product_type = 'cxc_subscription';
parent::__construct( $product );
}
}
}
?>
Adds the custom product type to the product type selector
<?php
add_filter( 'product_type_selector', 'cxc_add_cxc_subscription_product_type' );
function cxc_add_cxc_subscription_product_type( $types ){
$types[ 'cxc_subscription' ] = __( 'Cxc Subscription');
return $types;
}
?>
This code snippet specifically adds the custom product type to the product type selector. You may need to implement additional code to define the behavior, data fields, and display options for the “Cxc Subscription” product type if you want to create a fully functional custom product type.
Add custom functionality
<?php
add_action( 'admin_footer', 'cxc_subscription_custom_js_call_back' );
function cxc_subscription_custom_js_call_back() {
if ( 'product' != get_post_type() ) { return; }
?>
<script type='text/javascript'>
jQuery( document ).ready( function() {
jQuery( '.options_group.pricing' ).addClass( 'show_if_cxc_subscription' ).show();
jQuery( '.general_options' ).show();
});
</script>
<?php
}
add_filter( 'woocommerce_product_data_tabs', 'cxc_subscription_product_tab_call_back' );
function cxc_subscription_product_tab_call_back( $tabs) {
$tabs['cxc_subscription'] = array(
'label' => __( 'Cxc Subscription Tab', 'cxc-codexcoach' ),
'target' => 'cxc_subscription_product_options',
'class' => 'show_if_cxc_subscription_product',
);
return $tabs;
}
?>
This code is used to add custom functionality related to the “Cxc Subscription” product type in WooCommerce.
After adding this code, the JavaScript will execute on the product editing page, showing/hiding the necessary elements based on the product type, and the “Cxc Subscription Tab” will be added as a tab in the product data section.
Display custom product options
<?php
add_action( 'woocommerce_product_data_panels', 'cxc_custom_product_options_product_tab_content' );
function cxc_custom_product_options_product_tab_content() { ?>
<div id='cxc_subscription_product_options' class='panel woocommerce_options_panel'>
<div class='options_group'>
<?php
woocommerce_wp_checkbox( array(
'id' => '_enable_custom_product',
'label' => __( 'Enable Subscription'),
) );
woocommerce_wp_text_input(
array(
'id' => 'cxc_subscription_product_info',
'label' => __( 'Enter Subscription Details', 'cxc-codexcoach' ),
'placeholder' => '',
'desc_tip' => 'true',
'description' => __( 'Enter subscription details.', 'cxc-codexcoach' ),
'type' => 'text'
)
);
?>
</div>
</div>
<?php
}
?>
This code snippet adds a function called cxc_custom_product_options_product_tab_content
to display the content of the “Cxc Subscription” product tab.
Inside the function, a div element with the ID “cxc_subscription_product_options” is created, and it has the class “panel woocommerce_options_panel”. Within this div, you can add custom options for the “Cxc Subscription” product type.
After adding this code, the custom product options will be displayed within the “Cxc Subscription” product tab on the product editing page in WooCommerce.
Save Custom Product meta
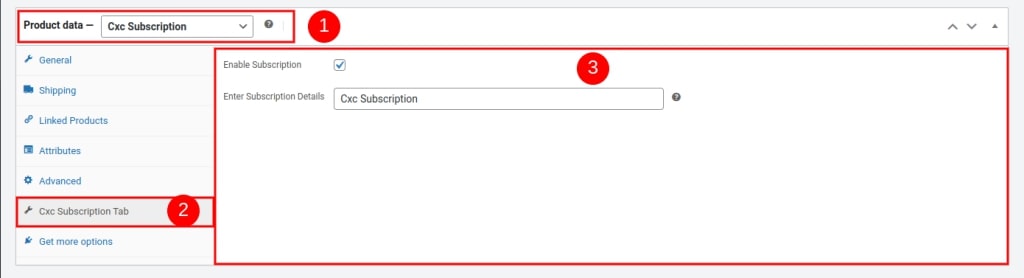
<?php
add_action( 'woocommerce_process_product_meta', 'save_cxc_subscription_product_settings' );
function save_cxc_subscription_product_settings( $post_id ){
$engrave_text_option = isset( $_POST['_enable_custom_product'] ) ? 'yes' : 'no';
update_post_meta($post_id, '_enable_custom_product', esc_attr( $engrave_text_option ));
$cxc_subscription_product_info = $_POST['cxc_subscription_product_info'];
if( !empty( $cxc_subscription_product_info ) ) {
update_post_meta( $post_id, 'cxc_subscription_product_info', esc_attr( $cxc_subscription_product_info ) );
}
}
?>
Final Result Display
<?php
add_action( 'woocommerce_single_product_summary', 'cxc_subscription_product_front' );
function cxc_subscription_product_front () {
global $product;
if ( is_object( $product ) && 'cxc_subscription' == $product->get_type() ) {
echo "<strong>Cxc Subscription Type: </strong>".( get_post_meta( $product->get_id(), 'cxc_subscription_product_info' )[0] );
}
}
?>
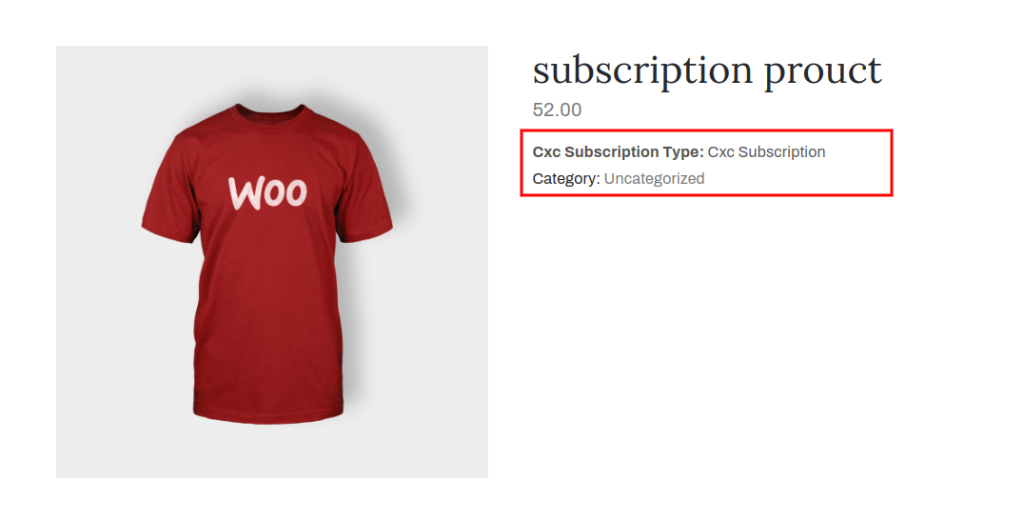
Creating a custom product type in WooCommerce provides the flexibility to tailor your online store to meet specific business needs and offer unique shopping experiences to your customers.
By following the steps outlined in this guide, you can extend the functionality of WooCommerce and create a fully functional custom product type programmatically.
With your newfound knowledge, you can explore further customization options, such as integrating additional functionality, extending the product display templates, or creating custom product calculations based on the specific product type.
Now that you understand how to create a custom product type in WooCommerce programmatically, you have the tools to build a dynamic and tailored shopping experience for your customers, setting your online store apart from the competition.