There are simply three steps required to add a new WooCommerce settings tab:
- Add your new tab to the list of currently active tabs.
- Make a setting array, then feed it to the output function.
- Send the same array to the function that saves the settings.
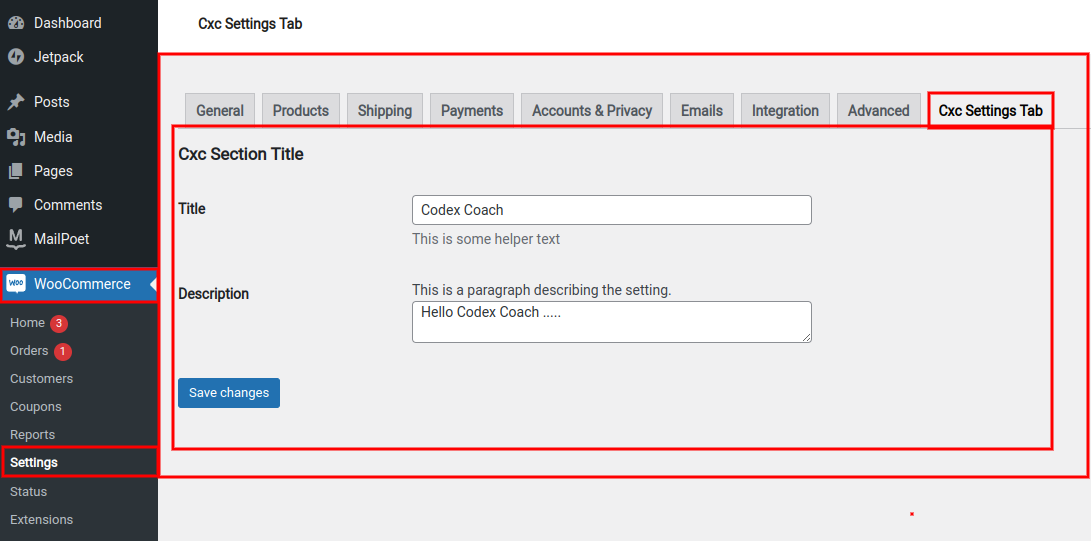
1) Add a New WooCommerce Settings Tab
The first step is to create a new settings tab. This is actually quite simple. Just one additional array item has to be added to the woocommerce_settings_tabs_array filter.
<?php
add_filter( 'woocommerce_settings_tabs_array', 'cxc_add_settings_tab', 50 );
function cxc_add_settings_tab( $settings_tabs ) {
$settings_tabs['cxc_settings_tabs'] = __( 'Cxc Settings Tab', 'cxc-codexcoach' );
return $settings_tabs;
}
?>
<?php
add_action( 'woocommerce_settings_tabs_cxc_settings_tabs', 'cxc_settings_tab' );
function cxc_settings_tab() {
woocommerce_admin_fields( cxc_get_settings() );
}
?>
2) Add Your Settings to the Settings Tab
<?php
function cxc_get_settings() {
$settings = array(
'section_title' => array(
'name' => __( 'Cxc Section Title', 'cxc-codexcoach' ),
'type' => 'title',
'desc' => '',
'id' => 'wc_cxc_settings_tabs_section_title'
),
'title' => array(
'name' => __( 'Title', 'cxc-codexcoach' ),
'type' => 'text',
'desc' => __( 'This is some helper text', 'cxc-codexcoach' ),
'id' => 'wc_cxc_settings_tabs_title'
),
'description' => array(
'name' => __( 'Description', 'cxc-codexcoach' ),
'type' => 'textarea',
'desc' => __( 'This is a paragraph describing the setting.', 'cxc-codexcoach' ),
'id' => 'wc_cxc_settings_tabs_description'
),
'section_end' => array(
'type' => 'sectionend',
'id' => 'wc_cxc_settings_tabs_section_end'
)
);
return apply_filters( 'wc_cxc_settings_tabs_settings', $settings );
}
?>
3) Save Your Settings
<?php
add_action( 'woocommerce_update_options_cxc_settings_tabs', 'cxc_update_settings' );
function cxc_update_settings() {
woocommerce_update_options( cxc_get_settings() );
}
?>