This tutorial was inspired by another one I came across, which advised creating WooCommerce coupons using the wp_insert_post() and update_post_meta() functions. Since WooCommerce 3.0, using objects is usually advised while working with its data. And coupons are not an exception.
We must utilize the WC_Coupon object to dynamically construct a coupon. We can utilize the object’s methods for coupon configuration once it has been created.
<?php
$coupon = new WC_Coupon();
$coupon->set_code( 'Cxc_Coupon_2023' ); // Add Coupon code here
$coupon->set_amount( 500 ); // You Can Add Discount amount here
$coupon->save();
?>
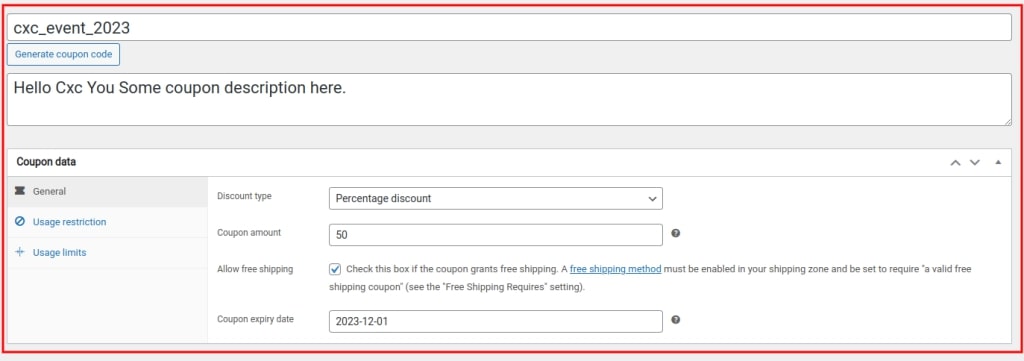
<?php
$coupon = new WC_Coupon();
$coupon->set_code( 'cxc_event_2023' );
$coupon->set_description( 'Hello Cxc You Some coupon description here.' );
// General tab
// discount type can be 'fixed_cart', 'percent' or 'fixed_product', defaults to 'fixed_cart'
$coupon->set_discount_type( 'percent' );
// discount amount
$coupon->set_amount( 50 );
// allow free shipping
$coupon->set_free_shipping( true );
// coupon expiry date
$coupon->set_date_expires( '01-12-2023' );
// Usage Restriction
// minimum spend
$coupon->set_minimum_amount( 5000 );
// maximum spend
$coupon->set_maximum_amount( 50000 );
// individual use only
$coupon->set_individual_use( true );
// exclude sale items
$coupon->set_exclude_sale_items( true );
// products ids
$coupon->set_product_ids( array( 181 ) );
// exclude products ids
$coupon->set_excluded_product_ids( array( 184, 193 ) );
// categories ids
$coupon->set_product_categories( array( 21 ) );
// exclude categories ids
$coupon->set_excluded_product_categories( array( 18, 22 ) );
// allowed emails
$coupon->set_email_restrictions(
array(
'[email protected]',
'[email protected]',
)
);
// Usage limit tab
// usage limit per coupon
$coupon->set_usage_limit( 500 );
// limit usage to X items
$coupon->set_limit_usage_to_x_items( 50 );
// usage limit per user
$coupon->set_usage_limit_per_user( 5 );
$coupon->save();
?>
Apply coupon programmatically to any product
Let’s start by learning how to use a coupon on any WooCommerce product. To accomplish this, we’ll run the script using the woocommerce_before_cart hook before the cart page loads. In addition, we’ll use the WooCommerce cart object to get the data we require to perform our validation prior to using the promo code we just made (cxc_coupon_2023).
Front-end pages have access to the WC()->cart object, which enables you to retrieve all data connected to the cart page. Therefore, add the following script to your child theme’s functions.php file:
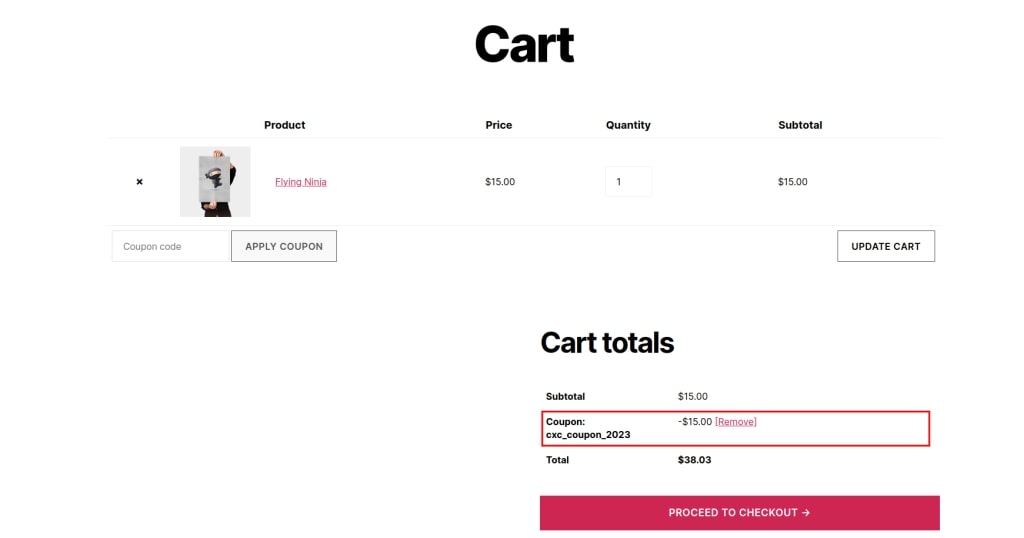
<?php
add_action( 'woocommerce_before_cart', 'cxc_apply_coupon_code_cart' );
function cxc_apply_coupon_code_cart() {
$cxc_coupon_2023 = 'cxc_coupon_2023';
if ( WC()->cart->has_discount( $cxc_coupon_2023 ) ){
return;
}
WC()->cart->apply_coupon( $cxc_coupon_2023 );
wc_print_notices();
}
?>
Apply WooCommerce coupons to specific product IDs
The ability to apply a coupon to particular WooCommerce product IDs is an additional intriguing choice. The script that follows can be used to accomplish that. Don’t forget to update the $products id() array with your product IDs.
<?php
add_action( 'woocommerce_before_cart', 'cxc_apply_matched_id_products' );
function cxc_apply_matched_id_products() {
// previously created coupon;
$cxc_coupon_2023 = 'cxc_coupon_2023';
// this is your product ID/s array
$product_ids = array( 181,184,193 );
// Apply coupon. Default is false
$cxc_apply = false;
// added to cart products loop
if( WC()->cart->get_cart() ){
foreach ( WC()->cart->get_cart() as $cart_item_key => $cart_item ) {
if ( in_array( $cart_item['product_id'], $product_ids ) ) {
// Id of product match
$cxc_apply = true;
break;
}
}
}
// apply & remove coupon
if( $cxc_apply == true ){
WC()->cart->apply_coupon( $cxc_coupon_2023 );
wc_print_notices();
} else {
WC()->cart->remove_coupons( sanitize_text_field( $cxc_coupon_2023 ));
wc_print_notices();
WC()->cart->calculate_totals();
}
}
?>
Apply coupon to the subtotal cart value
Now suppose you want to use a WooCommerce coupon when customers spend more than $50 at your business, as opposed to applying discounts based on the products.
This is a fantastic method to thank your customers and motivate them to make larger purchases from your website. As a result, the following feature only allows you to apply a discount when your cart’s sum is greater than $50.
<?php
add_action( 'woocommerce_before_cart','cxc_apply_coupon_cart_sub_total_values' );
function cxc_apply_coupon_cart_sub_total_values() {
// previously created coupon
$cxc_coupon_2023 = 'cxc_coupon_2023';
// get coupon
$current_coupon = WC()->cart->get_coupons();
// get Cart subtotal
$cart_sub_total = WC()->cart->subtotal;
// coupon has not been applied before && conditional is true
if( empty( $current_coupon ) && $cart_sub_total >= 50 ) {
// Apply coupon
WC()->cart->apply_coupon( $cxc_coupon_2023 );
wc_print_notices();
// coupon has been applied && conditional is false
} elseif( !empty( $current_coupon ) && $cart_sub_total < 50 ) {
WC()->cart->remove_coupons(sanitize_text_field($cxc_coupon_2023));
wc_print_notices();
WC()->cart->calculate_totals();
echo '<div class="woocommerce_message">Coupon was removed</div>';
// Conditional is FALSE && no coupon is applied
} else { // Do nothing
echo '<div class="woocommerce_message"> Unknown error</div>';
}
}
?>
Apply coupon cart total depending on the number of products on the cart page
Similar to that, you can use the script below to dynamically apply a WooCommerce coupon only when a certain quantity of items are in the cart. Say, for illustration, that you wish to offer a discount to clients who have four or more items in their shopping carts:
<?php
add_action( 'woocommerce_before_cart','cxc_apply_coupon_prodcut_by_count_call_back' );
function cxc_apply_coupon_prodcut_by_count_call_back(){
// previously created coupon
$cxc_coupon_2023 = 'cxc_coupon_2023';
// get coupon
$current_coupon = WC()->cart->get_coupons();
// get products count
$product_count =WC()->cart->get_cart_contents_count();
// coupon has not been applied before && conditional is true
if( empty( $current_coupon ) && $product_count >= 4 ) {
// Apply coupon
WC()->cart->apply_coupon( $cxc_coupon_2023 );
wc_print_notices();
// coupon has been applied && conditional is false
} elseif( !empty( $current_coupon ) && $product_count < 4 ) {
// remove coupon
WC()->cart->remove_coupons( sanitize_text_field( $cxc_coupon_2023 ) );
wc_print_notices();
WC()->cart->calculate_totals();
echo '<div class="woocommerce_message"> Coupon was removed</div>';
// Conditional is FALSE && no coupon is applied
} else { // Do nothing
echo '<div class="woocommerce_message"> Unknown error</div>';
}
}
?>
Auto-apply WooCommerce coupon code by URL
<?php
add_action( 'init', 'cxc_get_custom_coupon_code_to_sessions' );
function cxc_get_custom_coupon_code_to_sessions() {
if( isset( $_GET[ 'coupon_code' ] ) ) {
// Ensure that customer session is started
if( !WC()->session->has_session() )
WC()->session->set_customer_session_cookie(true);
// Check and register coupon code in a custom session variable
$coupon_code = WC()->session->get( 'coupon_code' );
if( empty( $coupon_code ) && isset( $_GET[ 'coupon_code' ] ) ) {
$coupon_code = esc_attr( $_GET[ 'coupon_code' ] );
WC()->session->set( 'coupon_code', $coupon_code ); // Set the coupon code in session
}
}
}
add_action( 'woocommerce_before_cart_table', 'cxc_apply_discount_to_carts', 10, 0 );
function cxc_apply_discount_to_carts() {
// Set coupon code
$coupon_code = WC()->session->get( 'coupon_code' );
if ( ! empty( $coupon_code ) && ! WC()->cart->has_discount( $coupon_code ) ){
WC()->cart->add_discount( $coupon_code ); // apply the coupon discount
WC()->session->__unset( 'coupon_code' ); // remove coupon code from session
}
}
?>
Conclusion
When establishing a coupon in WooCommerce, there are several choices accessible, and all of these parameters may be specified when creating a coupon programmatically.
If you have any questions or comments on the code, please leave them in the comments.
FAQ
How do I create a coupon code in WooCommerce?
Go to your WordPress Admin panel > Marketing > Coupons > Add coupon
How do I enable Coupons in WooCommerce?
Go to: WooCommerce > Settings > General > Enable coupons.
Tick the checkbox to Enable the use of coupon codes.
Save Changes.
How do you redeem discounts at self-service checkout?
You certainly can. All you have to do is scan them like the rest of your products. When you’re finished, look down near where you deposited the cash or coins to find a little slot where you may put the used coupons.
Saved me so much time, great posts as always.
This: WC()->cart->apply_coupon( $cxc_coupon_2023 ); took me a day to find, couldn’t find it anywhere in the docs! Thank you CodexCoach!!!