I’ll walk you through the process of generating products in WooCommerce using code in this article.
In the most recent WooCommerce versions, it is the most accurate method of creating products. Don’t use the wp_insert_post() and update_post_meta() functions, please. Yes, I do recall that product pricing are post meta and that products are WordPress custom post types, however we are not allowed to use those features because there is a little more than that and it might lead to errors.
We’ll use the CRUD objects introduced in WooCommerce 3.0 in this lesson (CRUD is an abbreviation of Create, Read, Update, Delete). Currently, we are interested in the following CRUD objects:
WC_Product_Simple
– for simple products,WC_Product_External
– for external products,WC_Product_Grouped
– for grouped products,WC_Product_Variable
– variable ones.
Simple Products
Let’s start by developing a straightforward product. I want you to consider what features a dead basic product should have. The product name, URL slug, price, and.. picture come first, right? Okay, perhaps we can describe a product along with its advantages!
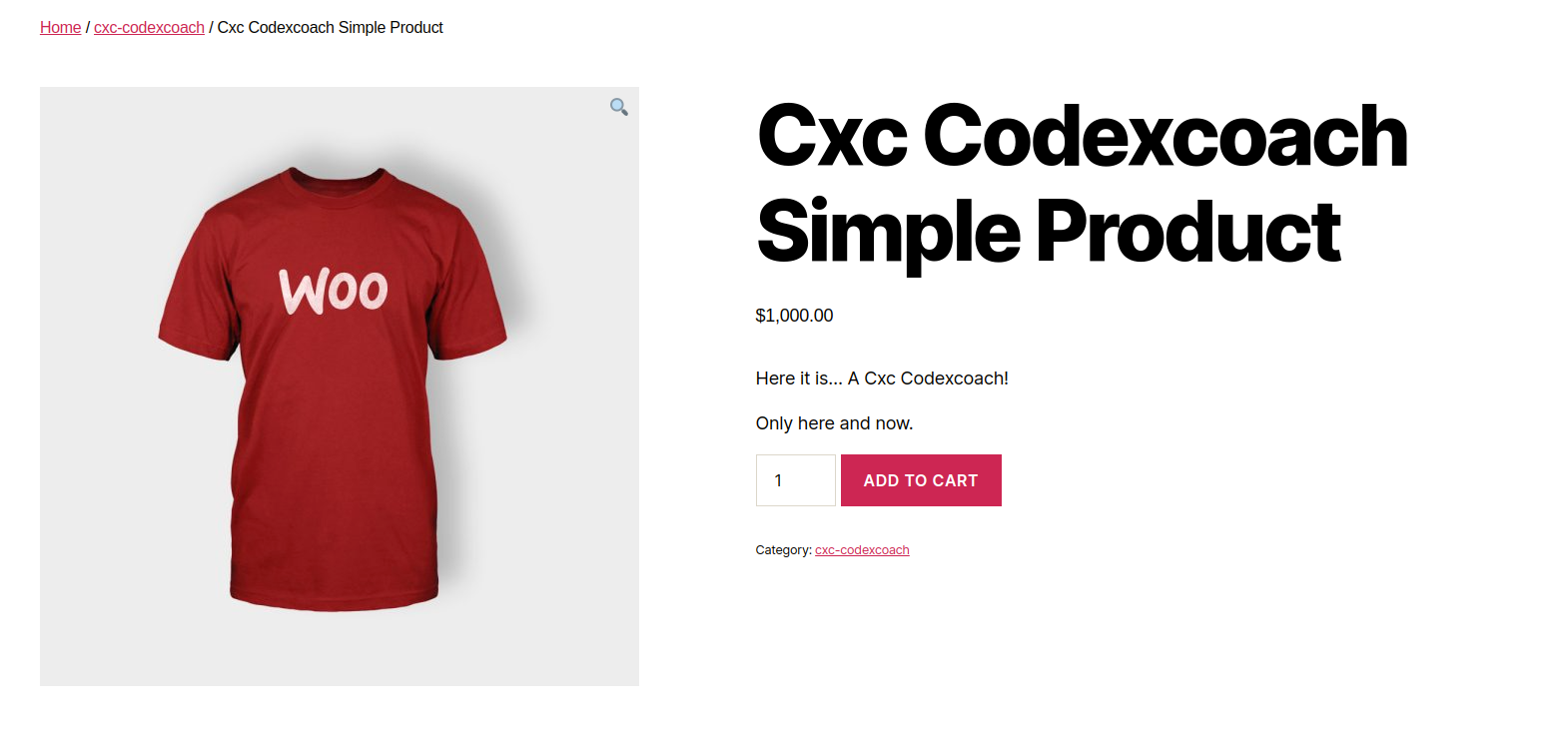
<?php
/* WC_Product_Simple Start */
// that's simple product object
$product = new WC_Product_Simple();
$product->set_name( 'Cxc Codexcoach Simple Product' ); // product title
$product->set_slug( 'cxc-codexcoach-simple-product' );
$product->set_regular_price( 1000.00 ); // in current shop currency
$product->set_short_description( '<p>Here it is... A Cxc Codexcoach!</p><p>Only here and now.</p>' );
// you can also add a full product description
// $product->set_description( 'long description here...' );
$product->set_image_id( 101 ); // attachmend ID should be here
// let's suppose that our 'Accessories' category has ID = 101
$product->set_category_ids( array( 101 ) );
// you can also use $product->set_tag_ids() for tags, brands etc
$product->save();
/* WC_Product_Simple End */
?>
You might also find the following techniques useful:
set_featured()
– passtrue
if you want this product to be marked as featured.set_gallery_image_ids()
– multiple image IDs can be passed as an array here.set_menu_order()
– manual product order as an integer.set_status()
– any post status here. Don’t want the product to be published? Setdraft
here.set_total_sales()
– product total sales can be passed here as an integer value.set_catalog_visibility()
– visibility in the catalog can be configured with this method,hidden
,visible
,search
andcatalog
values are accepted.update_meta_data()
– any product meta data, pass a pair of meta key and meta value as first and second parameters appropriately.
Products on Sale
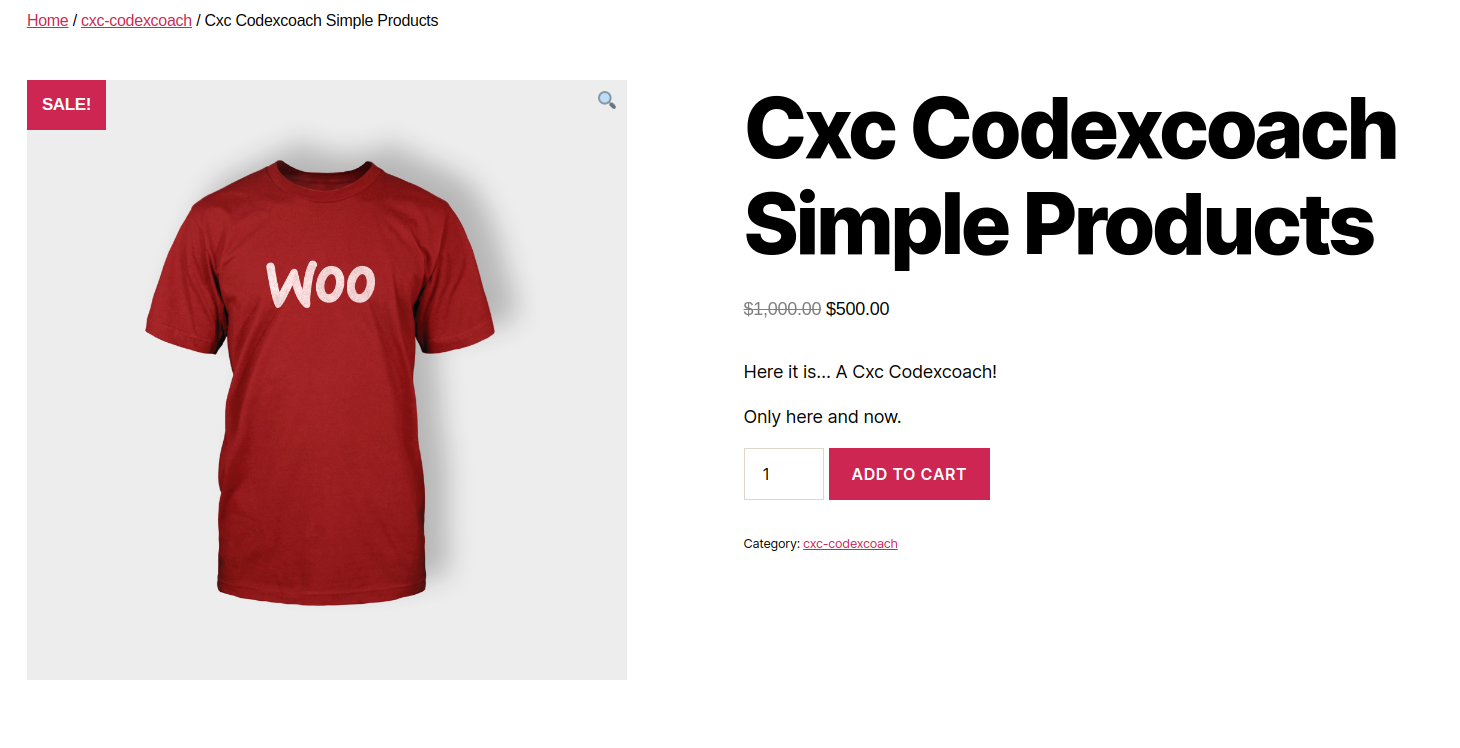
<?php
$product = new WC_Product_Simple();
$product->set_regular_price( 1000.00 );
$product->set_sale_price( 500.00 );
// Products on sale schedule
$product->set_date_on_sale_from( '2022-12-01' );
$product->set_date_on_sale_to( '2022-12-31' );
$product->save();
?>
Inventory Settings
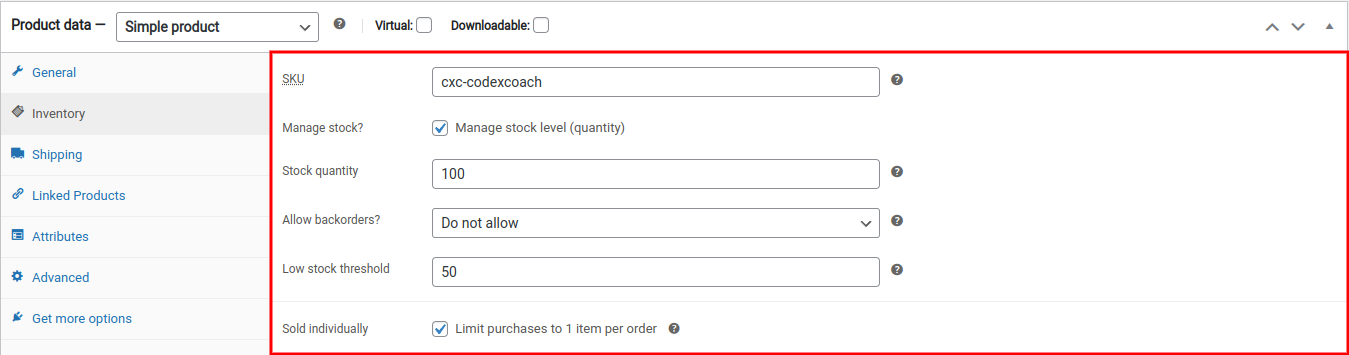
<?php
$product = new WC_Product_Simple();
$product->set_sku( 'cxc-codexcoach' ); // Should be unique
// You do not need it if you manage stock at product level (below)
$product->set_stock_status( 'instock' ); // 'instock', 'outofstock' or 'onbackorder'
// Stock management at product level
$product->set_manage_stock( true );
$product->set_stock_quantity( 100 );
$product->set_backorders( 'no' ); // 'yes', 'no' or 'notify'
$product->set_low_stock_amount( 50 );
$product->set_sold_individually( true );
$product->save();
?>
Dimensions and Shipping
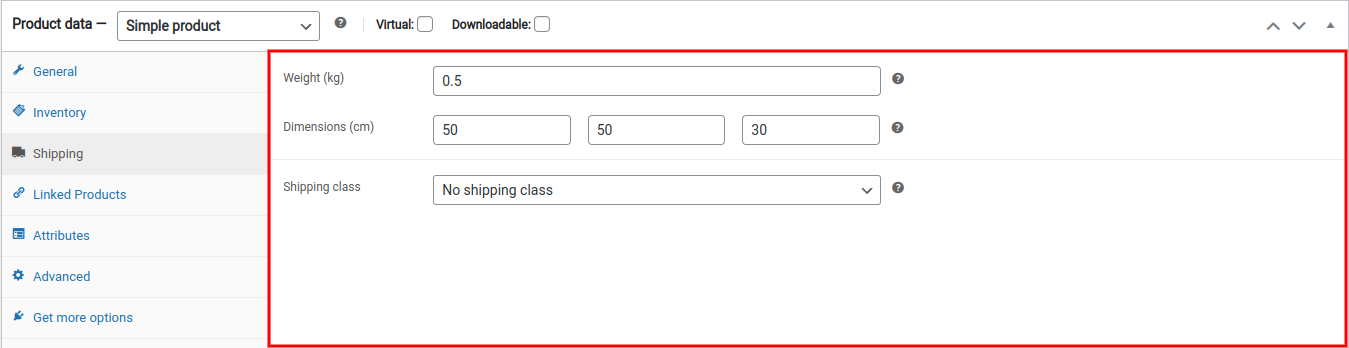
<?php
$product = new WC_Product_Simple();
$product->set_weight( 0.5 );
$product->set_length( 50 );
$product->set_width( 50 );
$product->set_height( 30 );
$product->set_shipping_class_id( 'hats-shipping' );
$product->save();
?>
Linked Products
The set_upsell_ids() and set_cross_sell_ids() functions each take an array of product IDs as input.
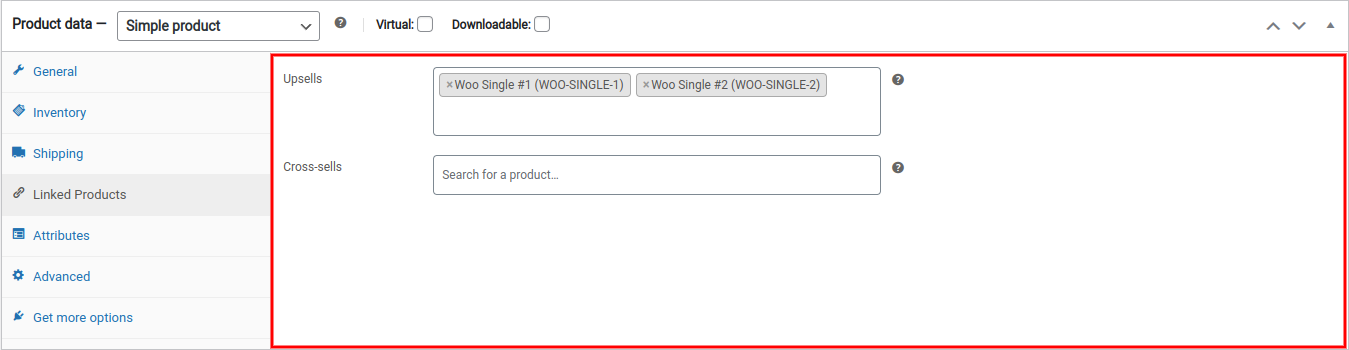
<?php
$product = new WC_Product_Simple();
$product->set_upsell_ids( array( 10, 11 ) );
// we can do the same for cross-sells
// $product->set_cross_sell_ids( array( 10, 11, 101, 201 ) );
$product->save();
?>
Products Attributes
First of all, I want to remind you that WooCommerce supports two different types of product attributes: custom Products > Attributes and predefined taxonomy-based attributes.
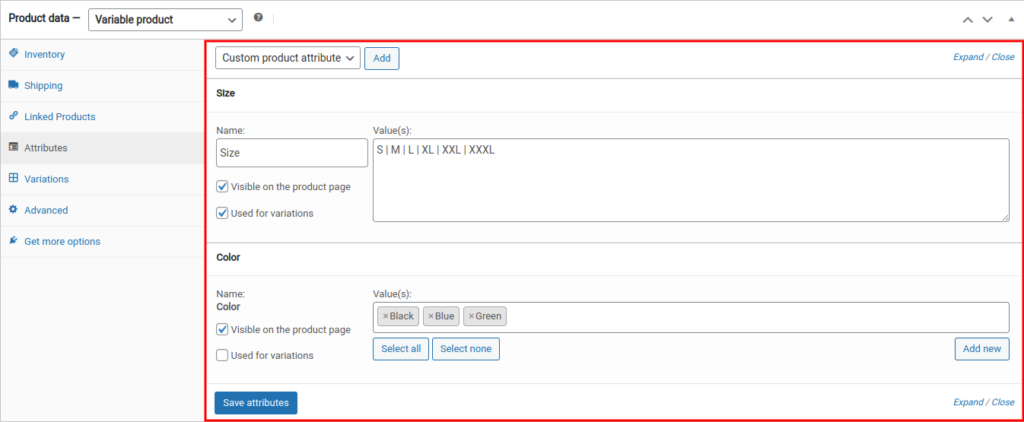
<?php
/* WC_Product_Variable Start */
// that's going to be an array of attributes we add to a product programmatically
$product = new WC_Product_Variable();
$product->set_name( 'Cxc Codexcoach Variable Product' );
$product->set_image_id( 90 );
$attributes = array();
$attributes_arr = array(
array(
'name' => 'Size',
'options' => array( 'S', 'M', 'L', 'XL', 'XXL', 'XXXL' ),
'position' => 0,
'visible' => true,
'variation' => true,
),
array(
'id' => wc_attribute_taxonomy_id_by_name( 'pa_color' ),
'name' => 'pa_color',
'options' => array( 25, 26, 27 ),
'position' => 1,
'visible' => true,
'variation' => false,
)
);
if( $attributes_arr ){
foreach( $attributes_arr as $value ) {
$attribute = new WC_Product_Attribute();
if( isset( $value['id'] ) ){
$attribute->set_id( $value['id'] );
}
$attribute->set_name( $value['name'] );
$attribute->set_options( $value['options'] );
$attribute->set_position( $value['position'] );
$attribute->set_visible( $value['visible'] );
$attribute->set_variation( $value['variation'] );
$attributes[] = $attribute;
}
}
$product->set_attributes( $attributes );
$product->save();
/* WC_Product_Variable End */
?>
Virtual and Downloadable products
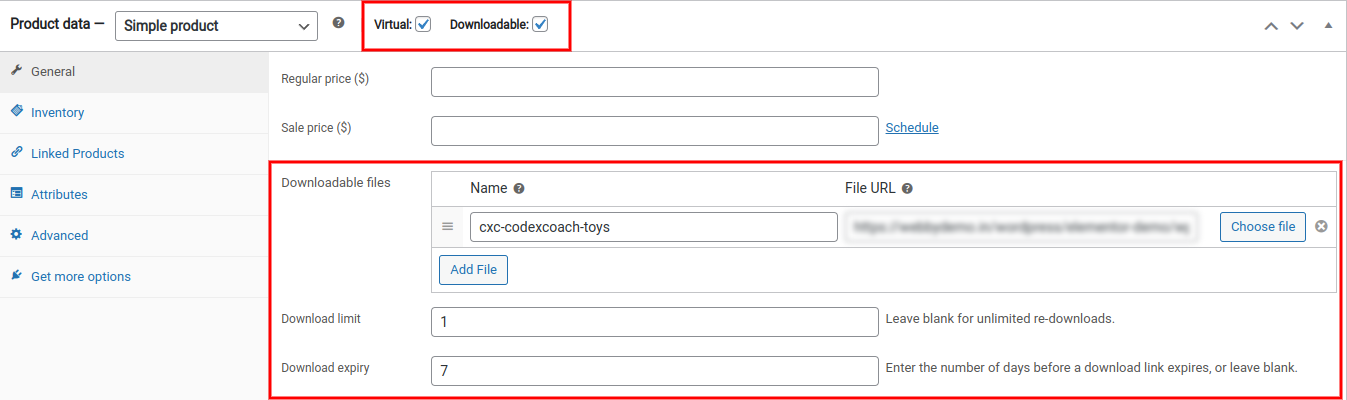
<?php
/* Virtual Product And Downloadable Product Start */
$product = new WC_Product_Simple();
$product->set_name( 'Cxc Codexcoach Virtual Product' );
// Virtual product : YES
$product->set_virtual( true );
// Downloadable product : YES
$product->set_downloadable( true );
$downloads = array();
// Creating a download with... yes, WC_Product_Download class
$download = new WC_Product_Download();
$file_url = wp_get_attachment_url( 101 ); // attachmend ID should be here
$download->set_name( 'cxc-codexcoach-toys' );
$download->set_id( md5( $file_url ) );
$download->set_file( $file_url );
$downloads[] = $download;
$product->set_downloads( $downloads );
$product->set_download_limit( 1 ); // can be downloaded only once
$product->set_download_expiry( 7 ); // expires in a week
$product->save();
/* Virtual Product And Downloadable Product End */
?>