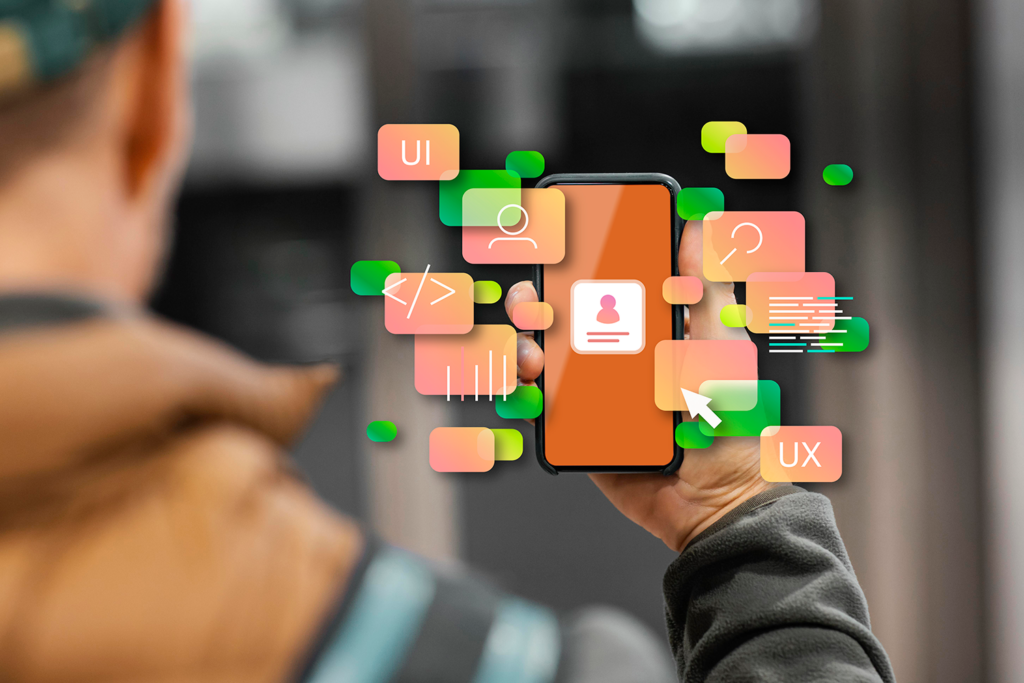
What is ARC in iOS development?
ARC stands for Automatic Reference Counting, and it’s like having a personal assistant who carefully manages the memory usage of your objects. It automatically frees up memory used by class instances when those instances are no longer needed.
Can you explain multithreading in iOS?
Multithreading is like having multiple workers in a coffee shop. Each worker can handle a different task simultaneously, making the whole operation more efficient. In iOS, this means your app can perform multiple operations at once without causing a traffic jam.
What’s the Singleton design pattern?
Imagine you have one key to your house that everyone shares. Similarly, Singleton is a design pattern that ensures a class has only one instance and provides a global point of access to it.
How does MVC help in iOS app development?
MVC, or Model-View-Controller, is like a play where each actor has a specific role. The Model is the brain, containing data and logic; the View is the stage design, presenting the UI; and the Controller is the director, managing the flow between the Model and the View.
What is dependency injection in iOS?
Dependency injection is like giving your friend exactly what they need to cook a meal instead of letting them find it themselves. In iOS, it’s a way to provide the objects that another object depends on (its dependencies) from the outside.
What is the Delegation pattern in iOS?
Think of delegation like asking a friend to pick up your coffee; you’re delegating the task. In iOS, delegation is a way for one object to delegate tasks to another object, without needing to know the details of how the task is carried out.
How do you manage app configuration in different environments?
Managing configurations is like having different outfits for various occasions. For iOS apps, you can use configuration files, which are like different sets of instructions, for development, testing, and production environments.
Explain the use of closures in Swift.
Closures in Swift are like customizable buttons on a remote. They let you write blocks of functionality that you can pass around and use in your code, just like setting a button to dim the lights or play your favorite show.
What is Core Data?
Core Data is like a librarian for your app’s data. It’s a framework that manages the objects your app needs to function, storing them efficiently, and retrieving them when you need them again.
Describe the use of optional in Swift.
Optionals are Swift’s way of saying something might be there, or it might not — like a mystery box. They are a safe way to handle values that could be nil, or in other words, nonexistent.
Explain Model-View-ViewModel (MVVM) in iOS.
MVVM is like a play with a backstage crew (ViewModel) that prepares everything the actors (View) need to perform their roles without interacting with the audience (Model). It promotes a more organized and efficient structure for your app, where the business logic and UI are separated, making your code cleaner and more manageable.
How do you handle data persistence?
For data that needs to stick around, like saving game scores or user preferences, you can use various methods. UserDefaults is like a notepad for quick notes, while more complex data might need a database like Core Data or SQLite, which are like filing cabinets for your app’s information.
What are some ways to ensure app security?
App security is like a bank vault for your data. Use HTTPS for secure communication, store sensitive information securely with Keychain, and employ proper authentication methods to keep the data safe from prying eyes.
How do you use Git for version control in iOS app development?
Git is like a time machine for your project’s code. It allows you to save snapshots of your work, branch out to try new things without affecting the main project and merge changes from different team members seamlessly.
Can you describe Test-Driven Development (TDD) in iOS?
TDD is like building a safety net before performing a trapeze act. You write tests for your functions before you even write the code to fulfill them, ensuring that as you code, you’re always meeting the established criteria.
What’s the difference between frame and bounds in iOS?
Think of frame as the size and location of a painting on a wall, while bounds are like the canvas size of the painting itself. In iOS, frame refers to a view’s size and position in its parent’s coordinate system, while bounds refer to the view’s own coordinate system.
How do you manage app states during interruptions like phone calls?
It’s like putting a bookmark in your book when someone calls you. iOS allows you to handle state transitions and interruptions by providing application lifecycle methods where you can save the app’s state and restore it once the interruption ends.
Explain Auto Layout in iOS.
Auto Layout is like a set of rules that tells your app how to size and position elements on the screen, so it looks good on any device, whether it’s a small iPhone SE or a large iPad Pro.
What are size classes in iOS development?
Size classes are like general size categories for device screens. They help you design adaptable layouts that provide a consistent experience on different devices, whether the user is on a compact iPhone or the expansive screen of an iPad.
How does iOS support internationalization and localization?
iOS supports internationalization and localization like a multilingual guide in a museum. It allows your app to support multiple languages and region-specific content, so users around the world can have a personalized experience.
What is a strong reference in Swift, and how does it relate to memory leaks?
A strong reference is like a firm handshake between two objects that says, “I need you to stick around.” It can lead to a memory leak if two objects strongly hold on to each other and won’t let go, preventing them from being discarded from memory.
Can you explain what a weak reference is?
A weak reference is more like a casual wave to an object. It tells the object, “I’m interested in you, but if you need to go, that’s okay.” It’s a way to reference an object without preventing it from being deallocated.
Describe the Responder Chain in iOS.
The Responder Chain is like a game of hot potato. When an event occurs, iOS passes it from one responder to the next until it finds the object that can handle the event.
What is Code Signing in iOS?
Code signing is like a wax seal on a letter; it verifies the identity of the app’s developer and ensures that the code hasn’t been tampered with since it was signed.
Explain what @objc is used for in Swift.
The @objc attribute is like a bridge that allows Swift methods to be visible to Objective-C, like translating instructions so that an old machine understands commands from a new operator.
What is an unowned reference in Swift?
An unowned reference is like a note to the compiler that says, “I’m expecting this to always be around while I’m here, but I won’t keep it alive.” It’s used when one instance has a shorter lifespan than another.
How do you use lazy properties in Swift?
A lazy property is like a snooze button on your alarm clock. It doesn’t get set up until you actually need it, conserving resources by only initializing an object when it’s first used.
Explain guard statements in Swift.
A guard statement acts as a bouncer at the entrance of a club. It checks if all conditions are met for the code to continue executing and if not, it sends you out early with an else clause.
What is a completion handler in iOS development?
A completion handler is like giving your friend a callback number to reach you once they’ve completed a task. In iOS, it’s a block of code that executes after a task is finished, allowing your app to continue with the necessary information.
Describe what @escaping closures are.
An @escaping closure is like a bird that leaves the nest; it can fly away to be used at a later time, escaping the function it was passed into and living on after the function returns.