Create a Meta Box
WordPress provides a function add_meta_box
to add meta boxes on whatever page we want. This function has the following syntax:
add_meta_box( string $id, string $title, callable $callback, string|array|WP_Screen $screen = null, string $context = 'advanced', string $priority = 'default', array $callback_args = null )
Where:
Name | Description |
$id (string) | Meta box ID (used in the 'id' attribute for the meta box). |
$title (string) | Title of the meta box. |
$callback (callable) | The function that fills the box with the desired content. The function should echo its output. |
$screen (string|array|WP_Screen) | The screen or screens on which to show the box (such as a post type, link , or comment ). |
$context (string) | The context within the screen where the boxes should display. Post edit screen contexts include ‘normal ‘, ‘side ‘, and ‘advanced ‘. Default value: ‘advanced ‘. |
$priority (string) | The priority within the context where the boxes should show (high , low ). Default value: high . |
$callback_args (array) | Data should be set as the $args property of the box array (which is the second parameter passed to your callback). Default value: null . |
We’ll create a meta box with the title “Cxc Nutrition facts” in the edit/create posts screen. Open the file “create-meta-boxes.php” and add the following snippet:
WordPress provides a add_meta_box function with the specific purpose to add a new Custom Meta Box. add_meta_box has to be called from inside a callback function that should be executed when the current page’s meta boxes are loaded. This task can be performed hooking the callback to the add_meta_box_{custom-post-type} action hook, as suggested in the Codex.
That being said, let’s add the following code to the main file of a plugin or a theme’s “create-meta-boxes.php“ file (keeping in mind that it’s always best to create a child theme instead of altering a “create-meta-boxes.php“ file):
<?php
/**
* Cxc Register Nutrition facts meta boxes.
*/
add_action( 'add_meta_boxes_post', 'cxc_post_add_meta_boxes' );
function cxc_post_add_meta_boxes() {
add_meta_box( 'cxc_nutrition_facts_meta_box', 'Cxc Nutrition facts', 'cxc_nutrition_facts_build_meta_box', 'post', 'normal', 'low' );
}
/**
* Cxc Nutrition facts Meta box display callback.
*
* @param WP_Post $post Current post object.
*/
function cxc_nutrition_facts_build_meta_box( $post ){
echo "Hello CodexCoach";
}
?>
Anything echoed in the function cxc_nutrition_facts_build_meta_box
will be displayed.

Add Custom Fields into a Meta Box
To add custom fields into a meta box, we need to modify the callback function to output form inputs. To shorten the code, let’s create a file "create-meta-boxes.php"
that contains the code of the form. Here is the snippet:
<?php
/**
* Cxc Nutrition facts Meta box display callback.
*
* @param WP_Post $post Current post object.
*/
function cxc_nutrition_facts_build_meta_box( $post ){
// make sure the form request comes from WordPress
wp_nonce_field( basename( __FILE__ ), 'cxc_nutrition_facts_meta_box_nonce' );
$cholesterol = get_post_meta( $post->ID, '_food_cholesterol', true );
// retrieve the _food_carbohydrates current value
$carbohydrates = get_post_meta( $post->ID, '_food_carbohydrates', true );
$vitamins = array( 'Vitamin A', 'Thiamin (B1)', 'Riboflavin (B2)', 'Niacin (B3)', 'Pantothenic Acid (B5)', 'Vitamin B6', 'Vitamin B12', 'Vitamin C', 'Vitamin D', 'Vitamin E', 'Vitamin K' );
// stores _food_vitamins array
$current_vitamins = ( get_post_meta( $post->ID, '_food_vitamins', true ) ) ? get_post_meta( $post->ID, '_food_vitamins', true ) : array();
?>
<div class='inside'>
<h3><?php _e( 'Cholesterol', 'food_example_plugin' ); ?></h3>
<p>
<input type="radio" name="cholesterol" value="0" <?php checked( $cholesterol, '0' ); ?> /> Yes<br />
<input type="radio" name="cholesterol" value="1" <?php checked( $cholesterol, '1' ); ?> /> No
</p>
<h3><?php _e( 'Carbohydrates', 'food_example_plugin' ); ?></h3>
<p>
<input type="text" name="carbohydrates" value="<?php echo $carbohydrates; ?>" />
</p>
<h3><?php _e( 'Vitamins', 'food_example_plugin' ); ?></h3>
<p>
<?php
foreach ( $vitamins as $vitamin ) {
?>
<input type="checkbox" name="vitamins[]" value="<?php echo $vitamin; ?>" <?php checked( ( in_array( $vitamin, $current_vitamins ) ) ? $vitamin : '', $vitamin ); ?> /><?php echo $vitamin; ?> <br />
<?php
}
?>
</p>
</div>
<?php
}
?>
Save the Custom Fields
<?php
/**
* Save meta box content.
*
* @param int $post_id Post ID
*/
add_action( 'save_post', 'cxc_food_save_meta_box_data' );
function cxc_food_save_meta_box_data( $post_id ){
// verify taxonomies meta box nonce
if ( !isset( $_POST['cxc_nutrition_facts_meta_box_nonce'] ) || !wp_verify_nonce( $_POST['cxc_nutrition_facts_meta_box_nonce'], basename( __FILE__ ) ) ){
return;
}
// return if autosave
if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ){
return;
}
// Check the user's permissions.
if ( ! current_user_can( 'edit_post', $post_id ) ){
return;
}
// store custom fields values
// cholesterol string
if ( isset( $_REQUEST['cholesterol'] ) ) {
update_post_meta( $post_id, '_food_cholesterol', sanitize_text_field( $_POST['cholesterol'] ) );
}
// store custom fields values
// carbohydrates string
if ( isset( $_REQUEST['carbohydrates'] ) ) {
update_post_meta( $post_id, '_food_carbohydrates', sanitize_text_field( $_POST['carbohydrates'] ) );
}
// store custom fields values
if( isset( $_POST['vitamins'] ) ){
$vitamins = (array) $_POST['vitamins'];
// sinitize array
$vitamins = array_map( 'sanitize_text_field', $vitamins );
// save data
update_post_meta( $post_id, '_food_vitamins', $vitamins );
} else {
// delete data
delete_post_meta( $post_id, '_food_vitamins' );
}
}
?>
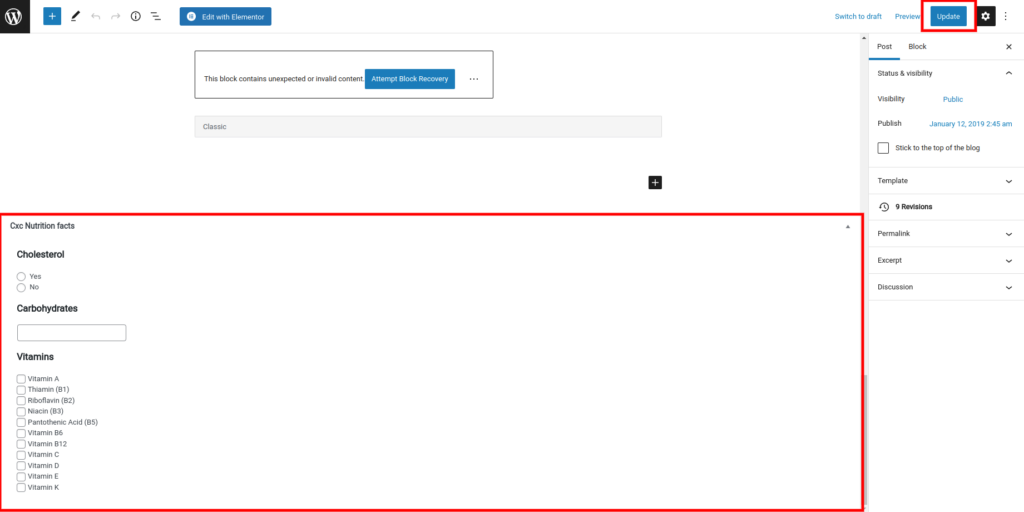