Alright, so you’re getting ready for a big interview in tech, and you’ve heard that data structure questions are a big deal, right? You’re spot on! Data structures are like the building blocks of coding; they help us organize and store data efficiently. Imagine you’re trying to find a book in a messy room it’d be a nightmare.
But if you have a well-organized bookshelf, you can find what you need quickly. That’s what data structures do in computer science.
Now, in interviews, you’ll encounter questions about arrays, linked lists, stacks, queues, trees, and graphs. They’ll ask you to solve problems, and you’ll have to discuss the time and space complexity of your solutions. It’s not just about knowing what these structures are but also understanding how to manipulate them efficiently.
So, buckle up! We’re diving deep into the world of data structure interview questions and answers. Whether you’re a beginner or looking to brush up on your skills, we’ve got you covered.
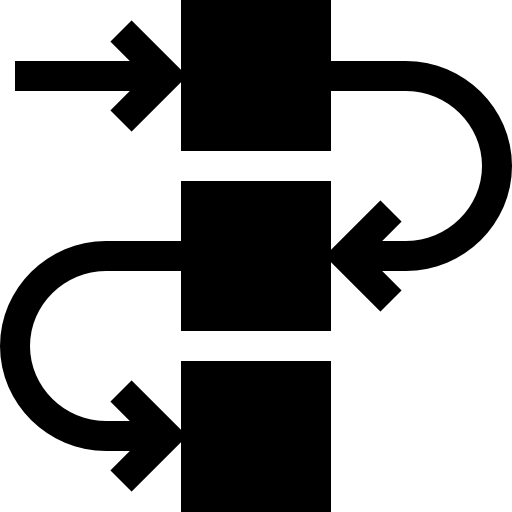
What is a Data Structure?
- A data structure is a specialized format for organizing, storing, and manipulating data on a computer. Data structures provide a means to manage large amounts of data efficiently.
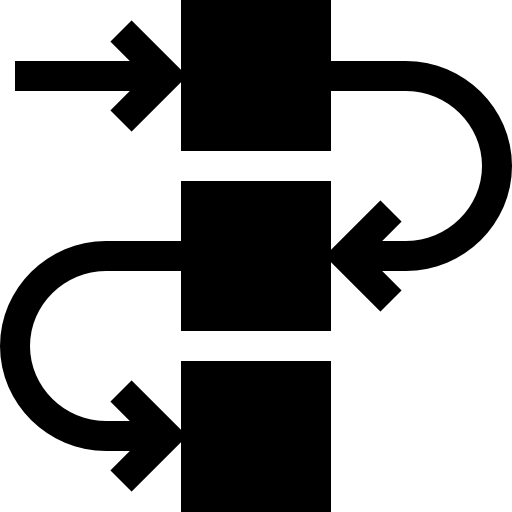
Explain the difference between an Array and a Linked List.
- An array is a contiguous block of memory that holds multiple elements of the same type. Elements in an array can be accessed in constant time using their index. However, the size of an array is fixed. A linked list is a collection of nodes, where each node contains data and a reference to the next node. Unlike arrays, linked lists are dynamic in size, but accessing elements requires traversing the list, which takes linear time.
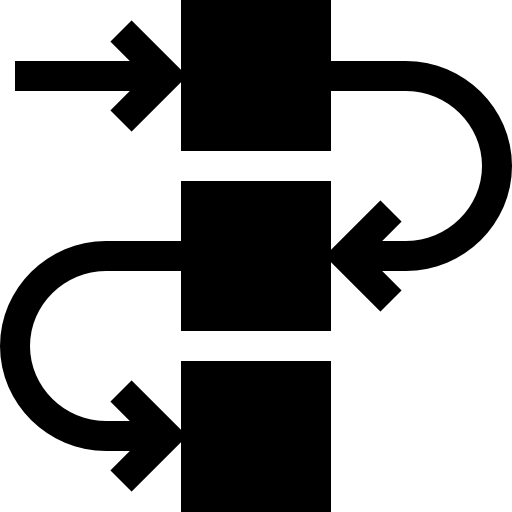
What is a Stack?
- A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. Elements can be pushed onto the top of the stack and popped off from the top.
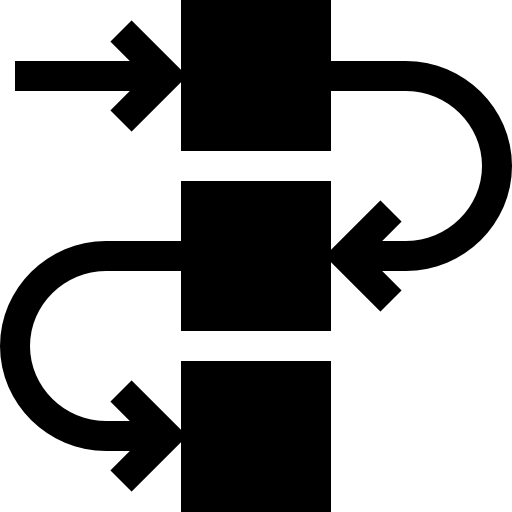
Explain the basic operations of a Queue.
- A queue is a linear data structure that follows the First In, First Out (FIFO) principle. The basic operations are enqueue, to insert an element at the rear, and dequeue, to remove an element from the front.
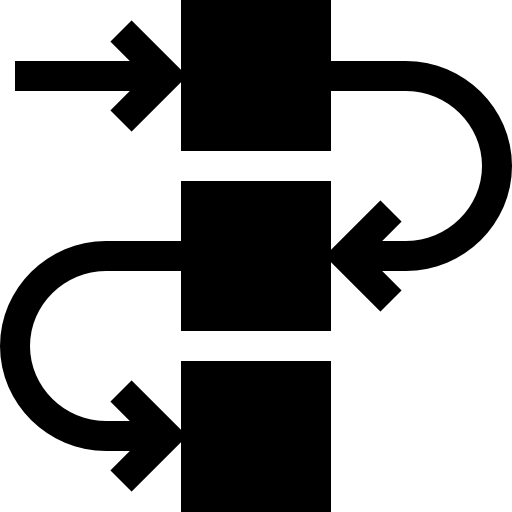
What is a Hash Table and how does it work?
- A hash table is a data structure that stores key-value pairs. It uses a hash function to compute an index into an array of buckets, where the value can be stored or retrieved.
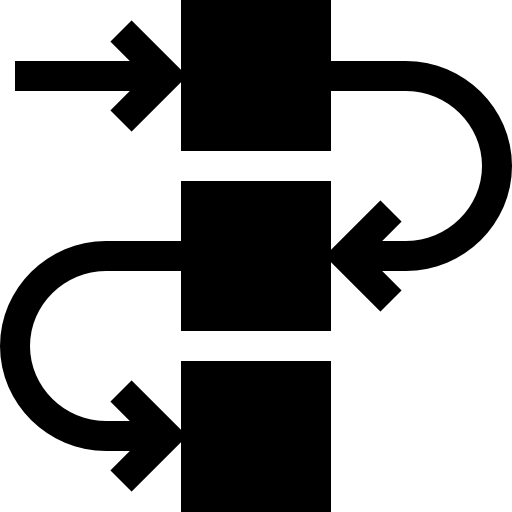
What is a Binary Tree?
- A binary tree is a hierarchical data structure where each node has at most two children, usually referred to as the “left child” and the “right child”.
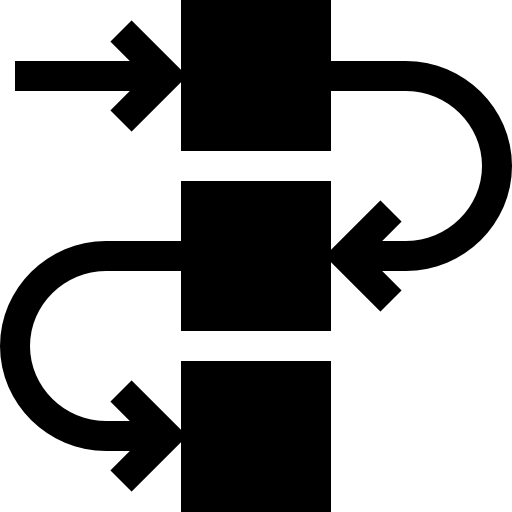
Describe Depth First Search (DFS) in graph traversal.
- Depth First Search is a graph traversal algorithm that starts at a source node and explores as far as possible along each branch before backtracking. It uses a stack or recursion for its implementation.
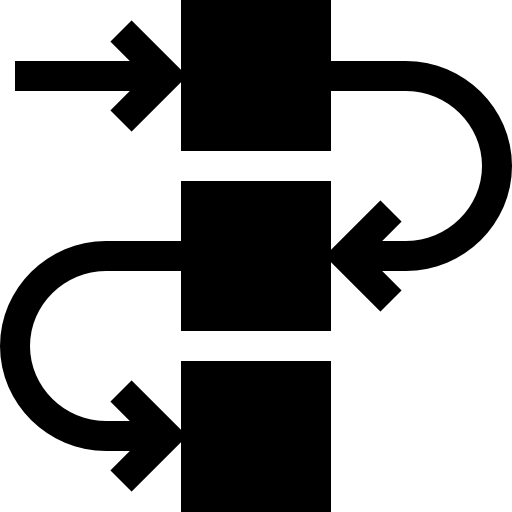
Explain the concept of Dynamic Programming.
- Dynamic Programming is an optimization technique that solves complex problems by breaking them down into smaller subproblems and storing the solutions to these subproblems to avoid redundant computations.
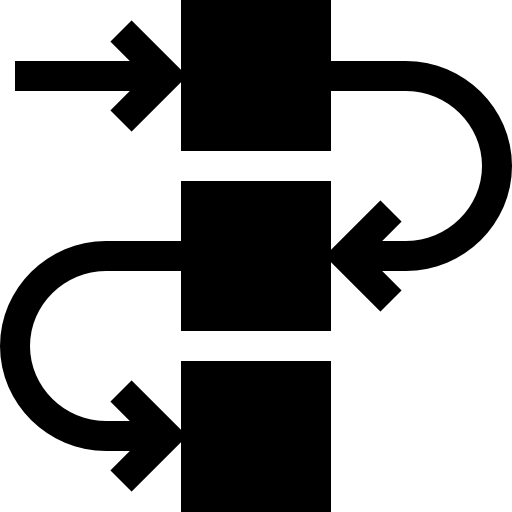
What is Big-O notation?
- Big-O notation is a mathematical notation used to describe the upper bound of an algorithm in the worst-case or average-case scenarios, with respect to the size of the input.
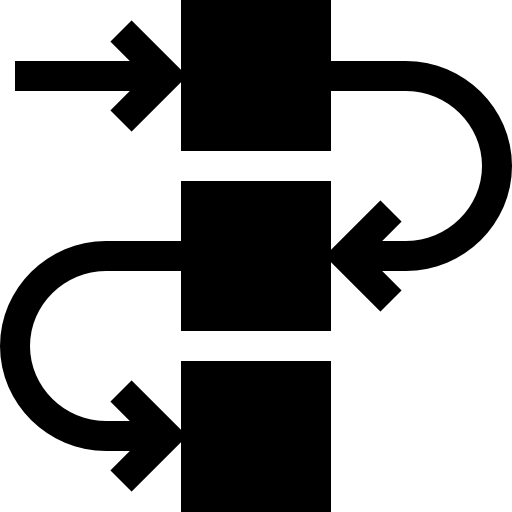
What is a Trie Data Structure?
- A Trie is a tree-like data structure used for storing a dynamic set of strings, where the keys are usually strings. Each node in a Trie has multiple children and represents a single character of a string.
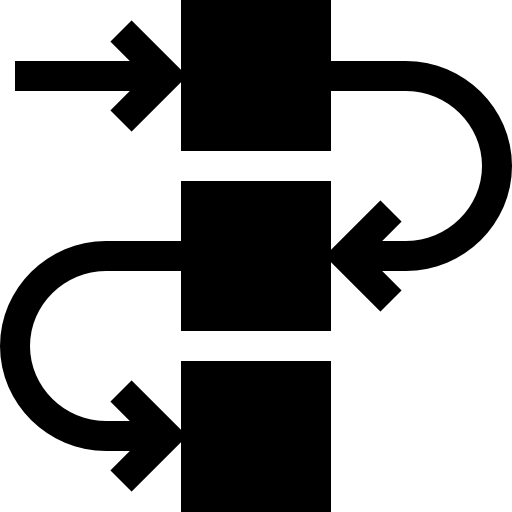
What is a Doubly Linked List?
- A doubly linked list is a type of linked list where each node contains a data part and two pointers. The first pointer points to the previous node in the list, and the second one points to the next node. This allows for traversal in both directions.
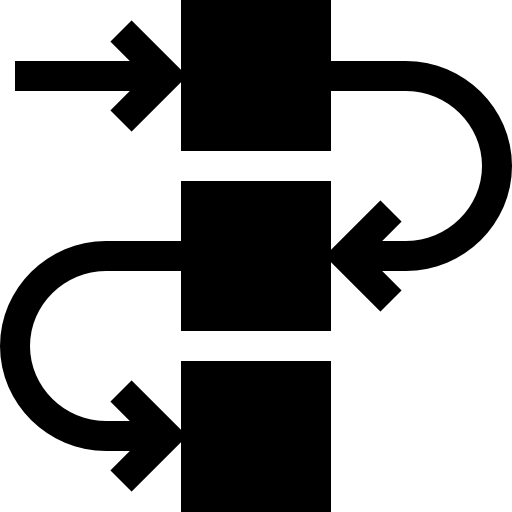
What is a Circular Queue?
- A circular queue is a data structure that uses a single, fixed-size array and two pointers to indicate the start and end positions, making the queue behave as if it were circular. When the rear reaches the end of the array, it starts from the beginning.
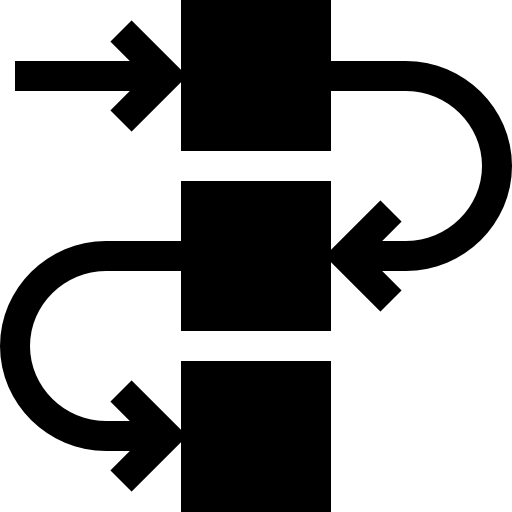
Explain Divide and Conquer algorithms.
- Divide and Conquer is an algorithmic paradigm that solves a problem by breaking it into smaller sub-problems, solving the sub-problems independently, and then combining their solutions to find the solution to the original problem.
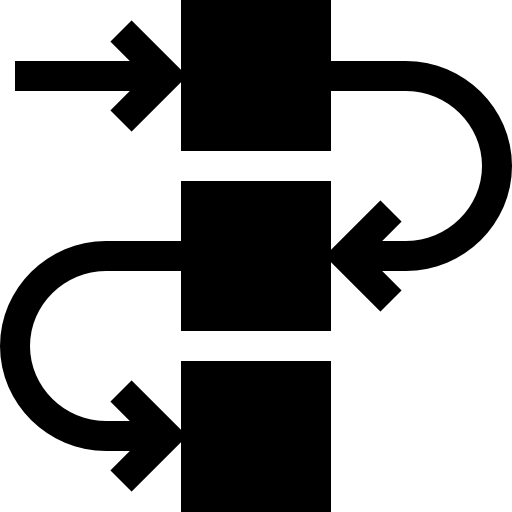
What is a Red-Black Tree?
- A Red-Black Tree is a type of self-balancing binary search tree where each node contains an extra bit for denoting the color of the node, either red or black. The tree satisfies certain properties to maintain the balance.
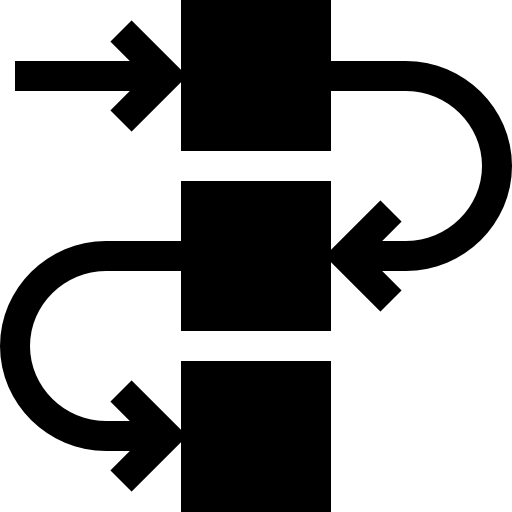
What is Memoization?
- Memoization is an optimization technique used primarily to speed up programs by storing the results of expensive function calls and reusing them when the same inputs occur again.
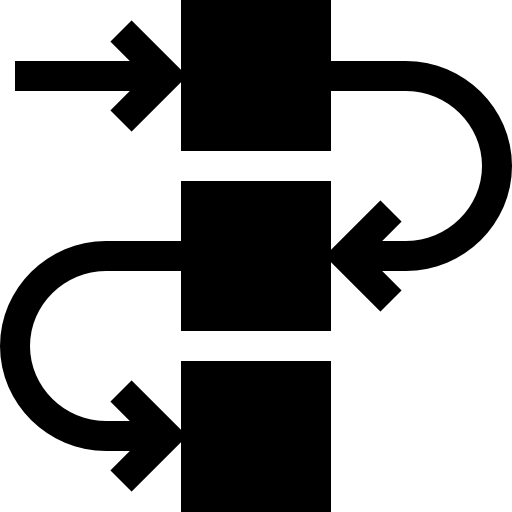
What are AVL Trees?
- AVL trees are a type of self-balancing binary search tree, where the height of the two child subtrees of every node differs by at most one. If at any point they differ by more than one, rebalancing is done to restore this property.
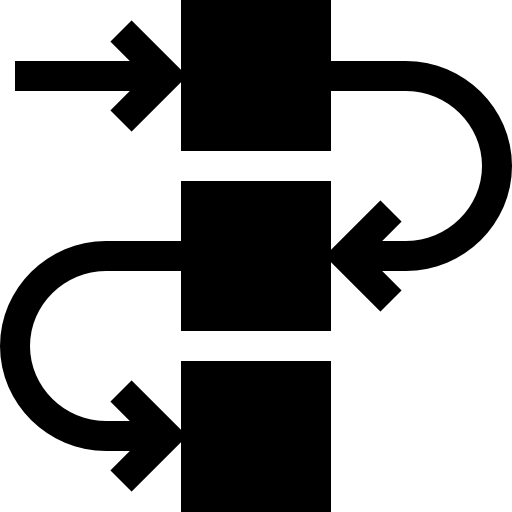
What is a Splay Tree?
- A splay tree is a self-adjusting binary search tree with the additional property that recently accessed elements are quick to access again. It performs basic operations like insertion, deletion, and lookup in amortized logarithmic time.
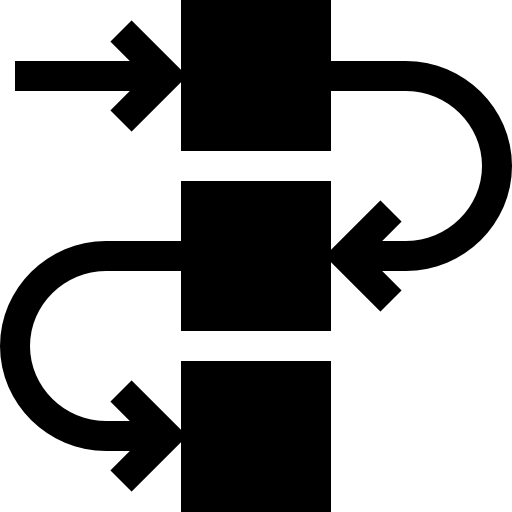
Explain Breadth First Search (BFS) in graph traversal.
- Breadth-first search is a graph traversal algorithm that explores all the vertices of a graph in breadth-first order, i.e., it explores all neighbors before going to the next level of neighbors. It uses a queue for its implementation.
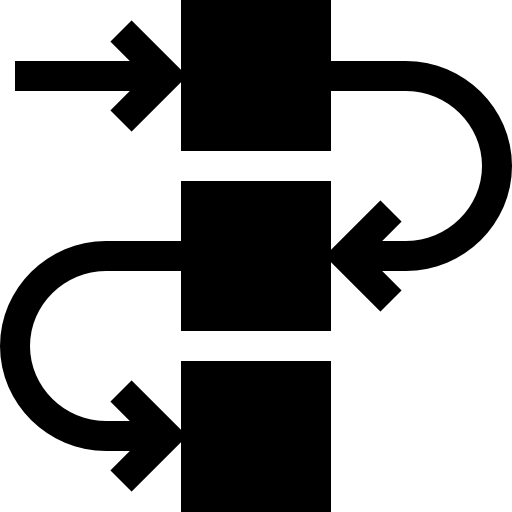
What is a Min-Heap and Max-Heap?
- Min-Heap and Max-Heap are specialized tree-based data structures that satisfy the heap property. In a Min-Heap, the key at the root must be minimum among all keys present in the heap, and the same property must be recursively true for all nodes. In a Max-Heap, the key at the root must be maximum among all keys.
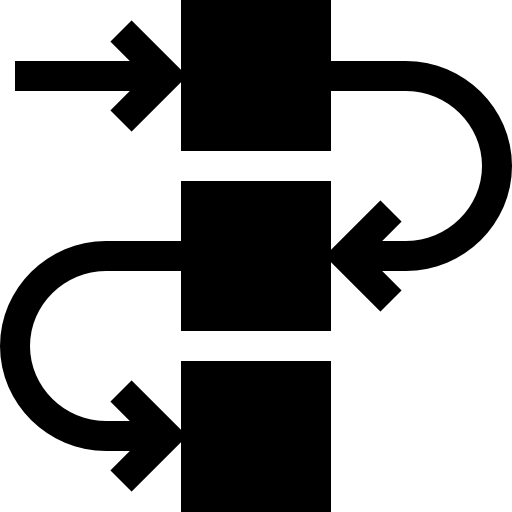
What is a Skip List?
- A skip list is a data structure that allows fast search within an ordered sequence of elements with O(log n) complexity. It does this by maintaining multiple layers of sub-lists, each of which skips over a fixed number of elements.
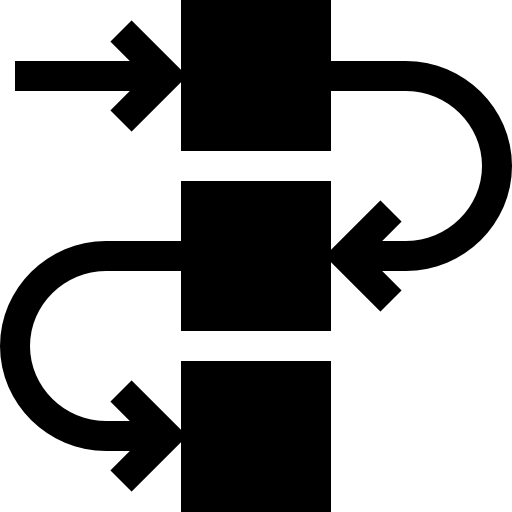
What is Graph Coloring?
- Graph Coloring is the assignment of labels, called colors, to the vertices of a graph in such a way that no two adjacent vertices have the same color.
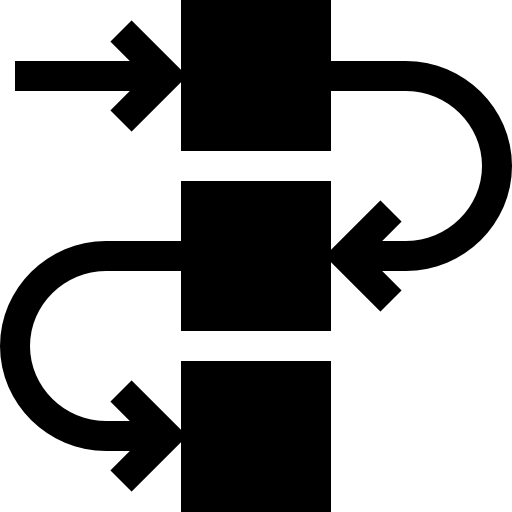
What is a Binary Search Algorithm?
- Binary search is an efficient algorithm for finding a target value within a sorted array. It reduces the problem size in half at each step and has a time complexity of O(log n).
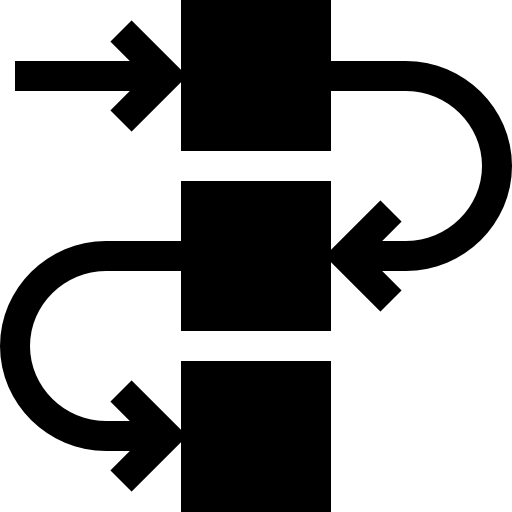
What is a Priority Queue?
- A priority queue is a data structure that stores elements with associated priorities. Elements are served based on their priority, not the order in which they arrive.
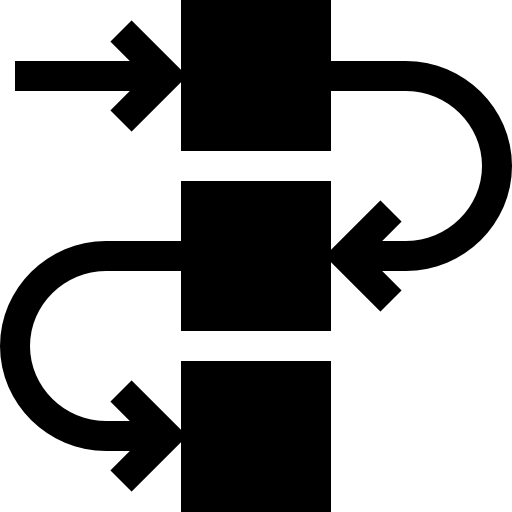
What is Bubble Sort?
- Bubble Sort is a simple sorting algorithm that works by repeatedly swapping adjacent elements if they are in the wrong order. It has a time complexity of O(n^2).
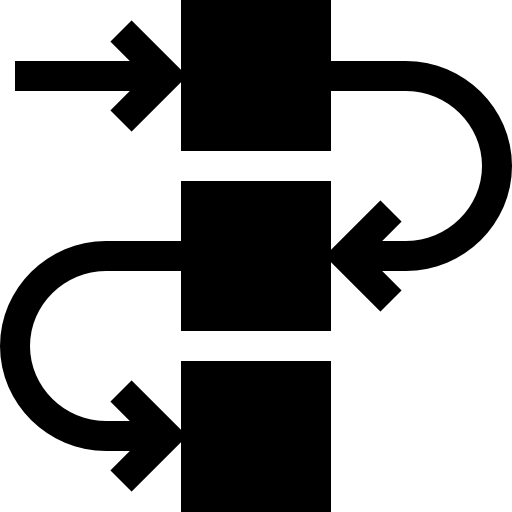
What are the types of Linked Lists?
- Linked lists can be categorized into Singly Linked Lists, Doubly Linked Lists, and Circular Linked Lists, each having different properties regarding node connectivity.
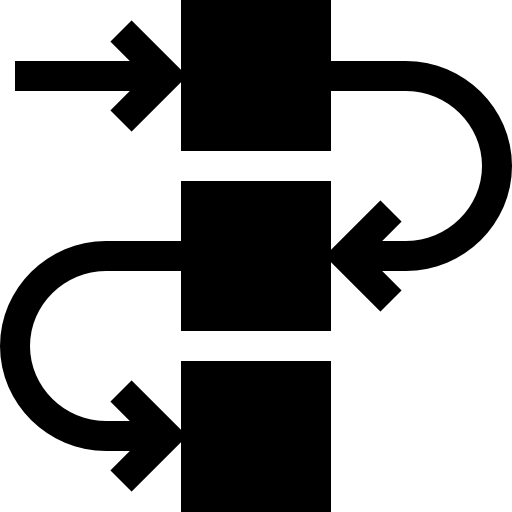
What are Hash Collisions and how are they resolved?
- A hash collision occurs when two different keys produce the same hash value. Resolutions include techniques like open addressing and separate chaining.
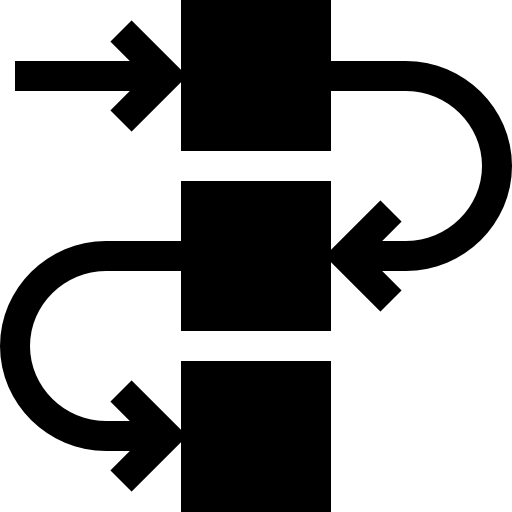
What is a K-D tree?
- A K-D tree, or k-dimensional tree, is a space-partitioning data structure useful for organizing points in a k-dimensional space.
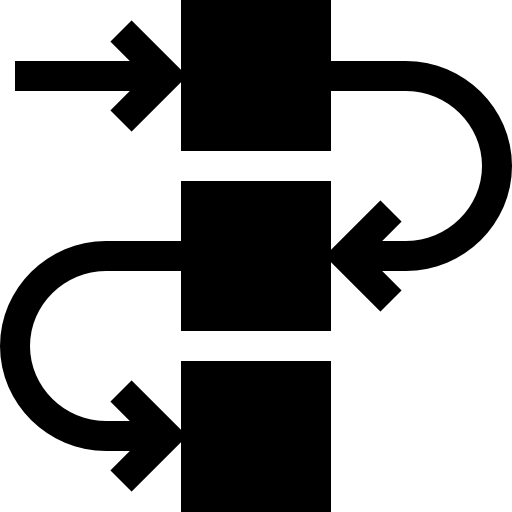
What is Inorder, Preorder, and Postorder tree traversal?
- These are methods to visit all the nodes in a tree. In Inorder traversal, the left subtree is visited first, then the root, and finally the right subtree. In Preorder, the root is visited first, then the left subtree, and finally the right subtree. In Postorder, both subtrees are visited before the root.
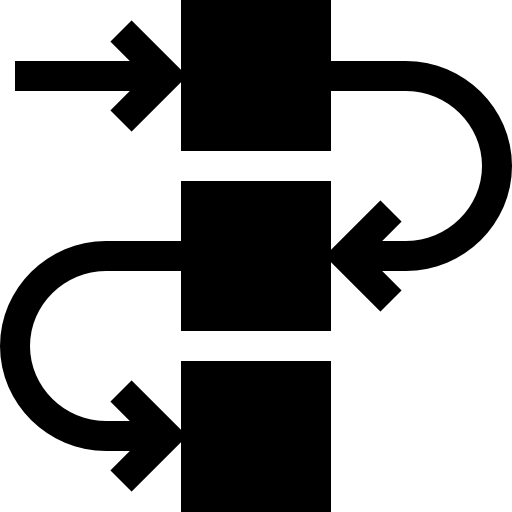
What is a Disjoint Set?
- A disjoint set is a data structure that keeps track of a set of elements partitioned into a number of disjoint, non-overlapping subsets.
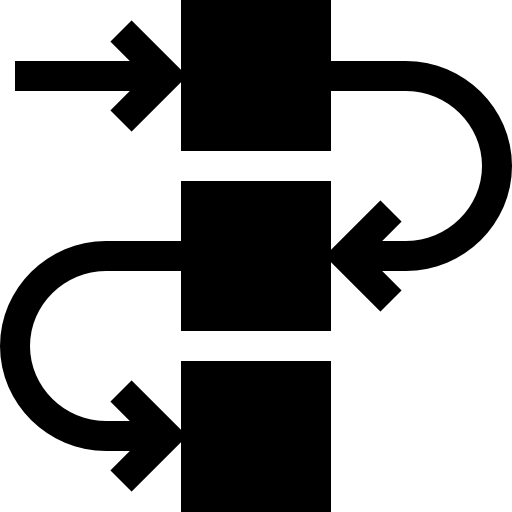
What is Dijkstra’s Algorithm?
- Dijkstra’s algorithm is used to find the shortest path from a source vertex to all other vertices in a weighted graph. It has a time complexity of O(V^2), but with a priority queue, it can be improved to O(V + E log V).
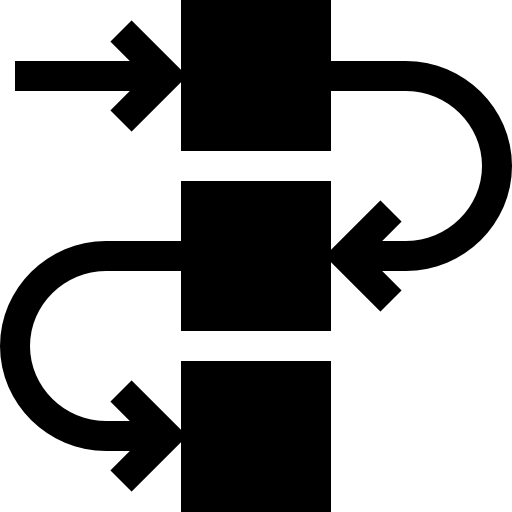
What is a B-tree?
- A B-tree is a self-balancing tree structure that maintains sorted data in a way that allows for efficient insertion, deletion, and search operations. It is commonly used in databases and filesystems.
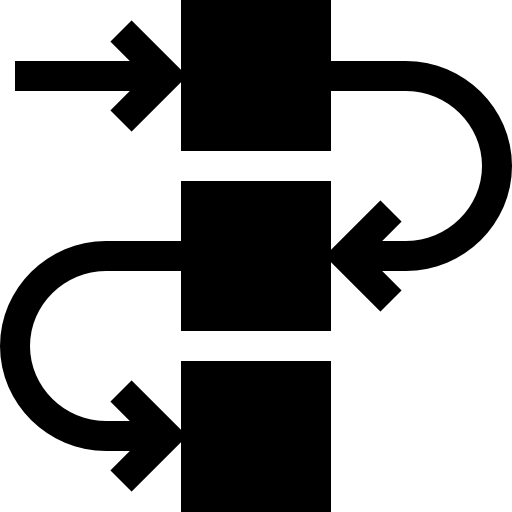
What is a Sparse Matrix?
- A sparse matrix is a matrix in which most of the elements are zero. Special data structures are used for their representation to save space.
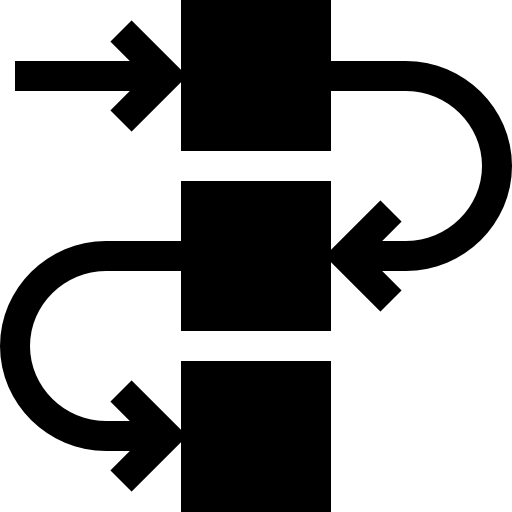
What are Suffix Trees?
- A suffix tree is a compressed trie containing all the suffixes of a given text. It’s often used in string-matching algorithms.
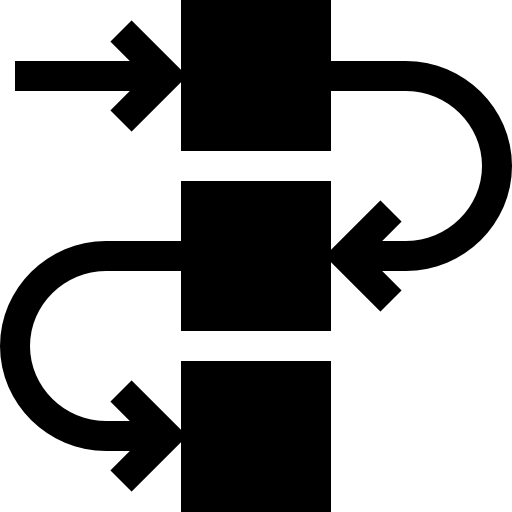
What is a Bloom Filter?
- A Bloom filter is a probabilistic data structure used to test whether an element is a member of a set. It may yield false positives but never false negatives.
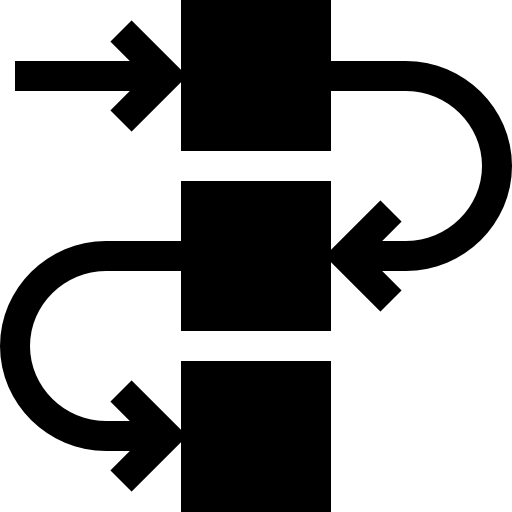
What is a Fenwick Tree?
- A Fenwick Tree, or Binary Indexed Tree (BIT), is a data structure that provides efficient methods for querying and updating prefix sums in an array.